Master CSV Writing in R: The Ultimate Step-by-Step Guide
Data manipulation proficiency with R hinges on the ability to efficiently manage data. The readr package offers streamlined functions; its utility is crucial for data import into R. One frequent task many data scientists face is to write csv in r. It is a vital process because CSV files serve as a universal data exchange format. RStudio, a popular IDE, provides an intuitive environment for executing commands, thus facilitating the smooth execution of write csv in r operations. This guide offers the essential steps to make this process seamless.
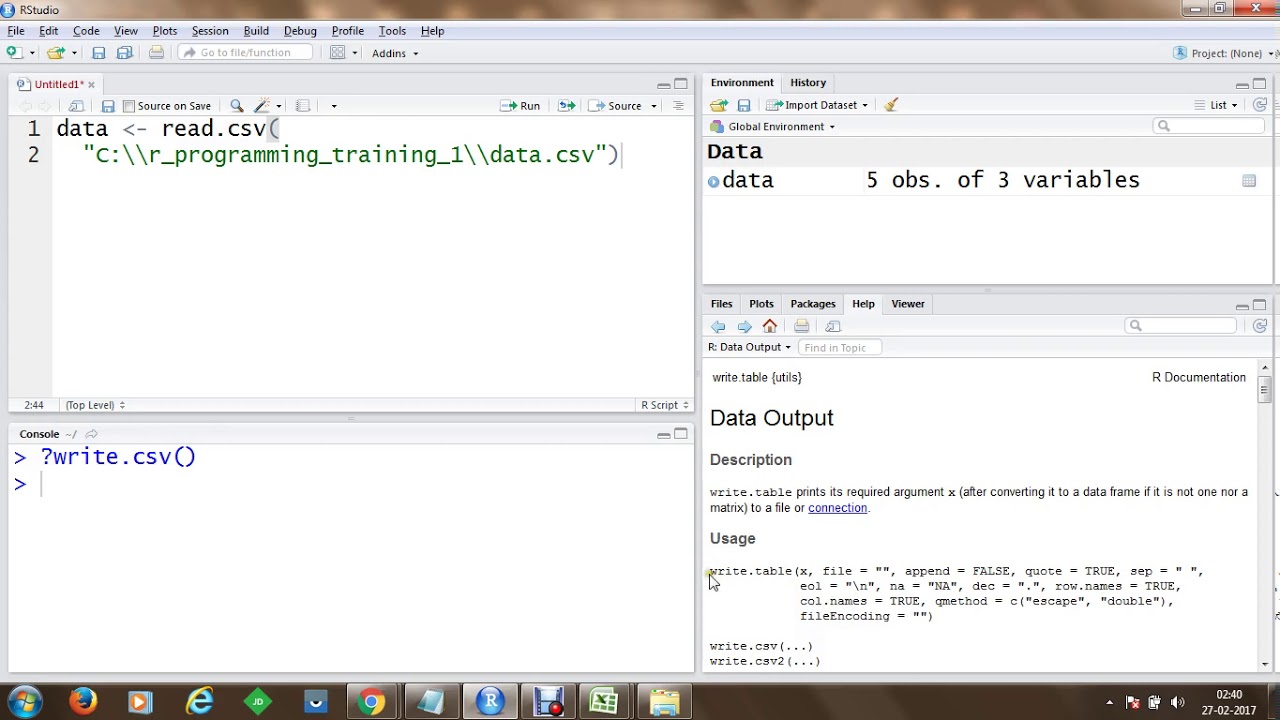
Image taken from the YouTube channel Hackveda Solutions , from the video titled R Programming: Write CSV Files in Rstudio .
The ability to efficiently write data to CSV (Comma Separated Values) files is a cornerstone of effective data analysis and manipulation. CSV files serve as a universally accessible format for storing tabular data, making them indispensable for data exchange, archiving, and integration with various software applications.
R, a powerful and versatile programming language and environment, has become the de facto standard for statistical computing and data science. Its extensive ecosystem of packages and built-in functionalities provides unparalleled capabilities for data wrangling, analysis, and visualization.
Why CSV Matters
CSV's simplicity is its strength. Its plain text nature ensures compatibility across diverse platforms and applications. This makes it ideal for sharing data between different systems, from databases to spreadsheets and statistical software.
CSV files facilitate:
- Data Portability: Seamlessly transfer data between applications.
- Archiving: Store data in a human-readable and easily accessible format.
- Collaboration: Share datasets with colleagues using various tools.
R: Your Data Manipulation Powerhouse
R's strength lies in its ability to transform, analyze, and visualize data. It offers a rich set of tools for data cleaning, transformation, and statistical modeling. R's open-source nature and extensive community support make it a cost-effective and constantly evolving solution for data professionals.
R empowers you to:
- Clean and Preprocess Data: Handle missing values, outliers, and inconsistencies.
- Perform Statistical Analysis: Conduct hypothesis testing, regression analysis, and more.
- Visualize Data: Create informative charts and graphs for data exploration and communication.
The Significance of Effective CSV Writing in R
While writing a CSV file might seem straightforward, mastering the nuances of this process is crucial for ensuring data integrity and reproducibility. Understanding the available options and best practices allows you to:
- Control Data Formatting: Customize delimiters, quotes, and encoding for specific requirements.
- Handle Data Types Correctly: Ensure that different data types (e.g., dates, factors) are written accurately.
- Optimize Performance: Choose the most efficient method for writing large datasets.
- Maintain Data Integrity: Prevent data loss or corruption during the writing process.
By understanding the tools and techniques for effectively writing CSV files in R, you unlock the full potential of this powerful combination, paving the way for robust and reproducible data analysis workflows.
The Significance of Effective CSV Writing in R
While writing a CSV file might seem straightforward, mastering the nuances of this process is crucial for reliable and reproducible data workflows. Understanding the intricacies of R's built-in functions empowers you to precisely control how your data is structured and stored. This ensures compatibility and facilitates seamless data exchange with other tools and platforms.
Writing CSV Files Using Base R: The write.csv() and write.table() Functions
R provides two fundamental functions for writing CSV files directly from your data frames: write.csv()
and write.table()
. These functions, part of the base R installation, offer a solid foundation for outputting data. Understanding their capabilities and differences is key to effectively managing your data output. Let's explore each function in detail.
Using write.csv()
The write.csv()
function is specifically designed for writing data frames to CSV files, with sensible defaults that align with common CSV conventions. It's a convenient and quick way to export your data for use in other applications.
Syntax and Basic Usage
The basic syntax for write.csv()
is straightforward:
write.csv(x, file, row.names = TRUE, ...)
Here, x
represents the data frame you want to write, and file
specifies the name and path of the CSV file to be created. The row.names
argument controls whether row names are included in the output.
Writing a Data Frame to CSV
Let's illustrate with a practical example. Suppose you have a data frame named mydata
:
mydata <- data.frame(
ID = 1:5,
Name = c("Alice", "Bob", "Charlie", "David", "Eve"),
Score = c(85, 92, 78, 89, 95)
)
To write this data frame to a CSV file named "mydata.csv", simply use:
write.csv(mydata, file = "my_data.csv")
This creates a CSV file in your current working directory.
Specifying File Paths
The file
argument is crucial. It determines where your CSV file is saved. You can use relative or absolute paths. A relative path is relative to your current working directory (e.g., "data/my_data.csv"). An absolute path specifies the complete location on your file system (e.g., "/Users/username/Documents/data/my_data.csv").
Working Directory Considerations
The working directory in R is the default location where R looks for files and saves output. You can determine your current working directory using getwd()
. To change it, use setwd("path/to/your/directory")
.
Setting the working directory ensures that R correctly interprets relative file paths when writing CSV files.
Controlling Column and Row Names
By default, write.csv()
includes column names in the first row of the CSV file and row names as a separate column. You can suppress writing row names by setting row.names = FALSE
:
write.csv(my_data, file = "my
_data.csv", row.names = FALSE)
Controlling these parameters is essential for achieving the desired CSV format.
Using write.table()
The write.table()
function is a more general function for writing data to files. It offers greater flexibility in customizing the output format. It can be used to write CSVs but requires more explicit specification of parameters.
Syntax and Basic Usage
The syntax for write.table()
is:
write.table(x, file = "", append = FALSE, quote = TRUE, sep = " ",
eol = "\n", na = "NA", dec = ".", row.names = TRUE,
col.names = TRUE, qmethod = c("escape", "double"),
fileEncoding = "")
While the function has many arguments, the key ones for writing CSVs are x
(the data), file
(the file path), sep
(the separator), row.names
, and col.names
.
Writing a Data Frame to CSV
To write our my_data
data frame to a CSV file using write.table()
, we need to explicitly set the separator to a comma:
write.table(mydata, file = "mydata_table.csv", sep = ",", row.names = FALSE)
Note that we also set row.names = FALSE
to avoid writing row names as a column.
Key Differences and When to Use Each
The main differences between write.csv()
and write.table()
are:
write.csv()
defaults to using a comma as a separator and includes column names.write.table()
requires you to explicitly specify the separator using thesep
argument.write.csv()
by default adds row names as a first column named "", whereaswrite.table()
uses standard numbering.write.csv()
is essentially a wrapper aroundwrite.table()
with preset parameters optimized for CSV output.
When to use which? Use write.csv()
when you need a quick and standard CSV output with comma separation and column names. Use write.table()
when you require more control over the output format. This is particularly useful when you need to use a different separator, such as tabs (\t
), for creating other types of delimited files.
Fine-Tuning Your Output: Essential Parameters and Options in Base R
Having covered the basic syntax and application of write.csv()
and write.table()
, it becomes clear that simply writing data is only the first step. To ensure your CSV files are truly compatible and usable across different systems and applications, you need to delve into the parameters that control their formatting. These parameters govern crucial aspects like how text is quoted, how fields are separated, and how character encoding is handled.
Controlling Quotes and Delimiters
The default settings for write.csv()
often suffice, but real-world data frequently demands more control. Imagine, for instance, data containing commas within a text field – without proper quoting, these commas would be misinterpreted as delimiters, corrupting your data structure.
Quote Characters
The quote
parameter in write.table()
(and implicitly used by write.csv()
) dictates how character strings are enclosed. By default, it's set to TRUE
, which means strings are enclosed in double quotes ("
).
However, you might want to change this behavior, perhaps to use single quotes ('
) or disable quoting altogether by setting quote = FALSE
. Disabling quotes should be done cautiously, only when you're absolutely certain that your data contains no delimiters within text fields.
Delimiters: Separating Fields
The sep
parameter defines the field separator. write.csv()
conveniently defaults to a comma (,
), making it a true CSV (Comma Separated Values) file.
write.table()
, on the other hand, defaults to a space (`). For creating tab-delimited files, often useful for specific applications, you would set
sep = "\t"`.
It's critical to choose a delimiter that never appears within your data to prevent parsing errors.
Here's a simple example demonstrating the use of quote
and sep
:
mydata <- data.frame(
ID = 1:3,
Description = c("Item, One", "Item Two", "Item Three")
)
write.table(mydata, file = "custom.csv", sep = ";", quote = TRUE, row.names = FALSE)
In this case, we've used a semicolon (;
) as the delimiter and retained double quotes around the "Description" field. Without the quotes, "Item, One" would be incorrectly parsed as two separate fields.
Specifying Encoding for Data Integrity
Character encoding is arguably one of the most critical, yet often overlooked, aspects of writing CSV files. Encoding defines how characters are represented as bytes, and failing to specify the correct encoding can lead to garbled or missing characters, especially when dealing with non-ASCII characters like accented letters, symbols, or characters from other languages.
The Importance of UTF-8
UTF-8 has emerged as the dominant character encoding for the web and data exchange. It's a variable-width encoding capable of representing virtually any character. Using UTF-8 is highly recommended for maximum compatibility and data integrity.
Setting the fileEncoding
Parameter
The fileEncoding
parameter in both write.csv()
and write.table()
allows you to specify the encoding of the output file. To ensure UTF-8 encoding, simply set fileEncoding = "UTF-8"
.
write.csv(mydata, file = "utf8_data.csv", fileEncoding = "UTF-8", row.names = FALSE)
Without explicitly setting the encoding, R will use the system's default encoding, which might not be UTF-8 and could lead to problems when the file is opened on different systems or with different software. Always be explicit about your encoding.
Dealing with Existing Encoding Issues
Sometimes, you might be working with data that already has encoding issues. Before writing to a CSV file, it's wise to ensure that the data is properly encoded in R.
You can use the iconv()
function to convert character strings between different encodings:
my_string <- "café" # Potentially problematic encoding
mystringutf8 <- iconv(my_string, from = "latin1", to = "UTF-8")
This example converts a string from Latin-1 (a common encoding) to UTF-8.
Examples of Parameter Settings and Their Impact
Let's consolidate these concepts with more illustrative examples.
Example 1: No Row Names, Tab-Delimited, UTF-8 Encoding
write.table(mydata, file = "tab_delimited.txt", sep = "\t", row.names = FALSE, fileEncoding = "UTF-8")
This creates a tab-delimited file without row names, encoded in UTF-8.
Example 2: Custom Delimiter, No Quotes, Handle NA values
mydata$Missing <- c(1,NA,3)
write.table(mydata, file = "custom_format.csv", sep = "|", quote = FALSE, na = "NULL", row.names = FALSE)
This example uses a pipe (|
) as the delimiter, disables quotes, and represents missing values (NA) as "NULL" in the output file.
Importance of Experimentation
The best way to fully grasp the impact of these parameters is to experiment. Create small data frames, modify the parameters, and inspect the resulting CSV files in a text editor. This hands-on approach will solidify your understanding and equip you to handle a wider range of CSV writing scenarios.
Mastering these parameters unlocks the true power of base R's CSV writing capabilities, enabling you to produce clean, consistent, and universally compatible data files.
Leveraging Packages for Enhanced CSV Writing: data.table and readr
While base R provides the foundational tools for writing CSV files, its performance can become a bottleneck, especially when dealing with larger datasets. Fortunately, R's vibrant ecosystem offers packages designed to overcome these limitations, notably data.table
and readr
. These packages not only enhance speed but also provide additional functionalities that streamline the CSV writing process.
Using the data.table
Package
The data.table
package is renowned for its efficiency in data manipulation, and its fwrite()
function is a game-changer for writing large CSV files.
The data.table
package extends the functionality of data frames, enabling faster data aggregation, manipulation, and writing. Its optimized algorithms and efficient memory management contribute to its superior performance.
Harnessing fwrite()
for Speed
The fwrite()
function within data.table
is specifically designed for high-speed file writing. Its syntax is straightforward:
library(data.table)
fwrite(my_data, "output.csv")
fwrite()
automatically detects the data type of each column and optimizes the writing process accordingly. This reduces overhead and leads to significant speed improvements compared to base R functions.
The key advantage of fwrite()
lies in its ability to handle large datasets with remarkable speed, making it an ideal choice when performance is critical.
Speed Comparison: fwrite()
vs. Base R
The performance difference between fwrite()
and base R functions becomes evident when dealing with large datasets. Benchmarking studies consistently demonstrate that fwrite()
can write CSV files several times faster than write.csv()
or write.table()
.
For instance, consider writing a data frame with millions of rows. fwrite()
can complete the task in a fraction of the time required by base R functions. This efficiency translates to significant time savings, especially in data-intensive workflows.
Using the readr
Package
The readr
package, part of the tidyverse ecosystem, offers a suite of functions for fast and efficient file reading and writing.
readr
focuses on providing a consistent and user-friendly interface for working with tabular data. Its functions are designed to be both fast and reliable.
write_csv()
: A Modern Alternative
The writecsv()
function in readr
offers a modern alternative to base R's write.csv()
. Its syntax is intuitive:
library(readr)
writecsv(my_data, "output.csv")
write_csv()
automatically handles quoting and delimiting, making it easy to produce well-formatted CSV files. It also supports writing directly to compressed files, such as gzipped CSVs.
Performance and Features of write_csv()
_csv()
While perhaps not quite as blistering as fwrite()
, write_csv()
still provides a performance boost compared to base R, especially when dealing with larger datasets.
One of its key features is its consistent handling of character encoding, ensuring that your data is written correctly regardless of the system or application used to read the file.
write
_csv()
is a good balance of speed, features, and ease of use making it a solid choice for many CSV writing tasks.Furthermore, write_csv()
is designed to seamlessly integrate with other tidyverse packages, making it a natural choice for users already familiar with this ecosystem.
Leveraging packages like data.table
and readr
significantly accelerates CSV writing, providing a notable advantage over base R functions, particularly for handling extensive datasets. But speed is just one piece of the puzzle. Ensuring data integrity, accuracy, and reproducibility requires careful consideration of various best practices.
Best Practices for Robust CSV Writing: Data Handling and Efficiency
Writing robust CSV files involves more than just executing a function; it requires a thoughtful approach to data handling, efficient processing, and diligent validation. Let’s explore key practices that contribute to creating reliable and reproducible CSV outputs.
Handling Different Data Types
CSV files, by their nature, are text-based. This means that all data types must be converted to character strings before being written. The way R handles this conversion can significantly impact the final output.
Dates: Dates are often stored internally as numerical values representing the number of days since a specific origin. When writing dates to CSV, it's essential to format them appropriately using functions like format()
or specifying the date
argument in fwrite()
or write_csv()
. Consistent formatting ensures that dates are interpreted correctly when the CSV is read back into R or another application.
Factors: Factors represent categorical variables with predefined levels. By default, R might write factors as their underlying integer representation. To preserve the categorical nature of the data, convert factors to character strings using as.character()
before writing to CSV.
Characters: While character strings are inherently compatible with CSV format, pay attention to character encoding. Using the correct encoding (e.g., UTF-8) ensures that special characters, accents, and symbols are represented accurately, avoiding data corruption.
Managing Missing Values (NAs)
Missing data is a common occurrence in real-world datasets. R represents missing values as NA
. When writing to CSV, the default behavior is to represent NA
as "NA".
Consider whether this representation is suitable for your needs. Some applications might interpret "NA" as a literal string rather than a missing value.
You can control how missing values are represented using the na
argument in functions like write.csv()
and fwrite()
. Common alternatives include leaving the cell empty ("") or using a specific placeholder value (e.g., "NULL", "-999"). Choose a representation that is consistent with your data context and the requirements of the system that will be reading the CSV file.
It’s also good practice to document your choice of missing value representation in a separate metadata file or README.
Optimizing for Large Datasets
Writing large datasets to CSV can be memory-intensive and time-consuming. Here are some tips for optimizing the process:
-
Use Efficient Packages: As discussed earlier,
data.table
andreadr
are significantly faster than base R functions for writing large CSV files. -
Avoid Unnecessary Conversions: Minimize data type conversions within your script, especially within loops. Perform conversions upfront whenever possible.
-
Control Memory Usage: If you encounter memory issues, consider writing the data in chunks. Divide your data frame into smaller subsets and write each subset to a separate CSV file or append them to the same file. Be cautious of opening and closing the file repeatedly, however, as that can be slower than writing to memory.
-
Monitor Performance: Use benchmarking tools to compare the performance of different approaches and identify bottlenecks in your code. The
microbenchmark
package is a great option.
Ensuring Data Integrity and Reproducibility
Data integrity and reproducibility are paramount for reliable data analysis. Here are strategies to promote these qualities:
-
Version Control: Use a version control system like Git to track changes to your R scripts and data files. This allows you to revert to previous versions if necessary and provides a clear audit trail of modifications.
-
Data Validation: Implement data validation checks to ensure that the data written to CSV meets your expectations. For example, verify that dates fall within a valid range, that numerical values are within acceptable bounds, and that categorical variables have valid levels.
-
Document Your Process: Maintain clear and comprehensive documentation of your CSV writing process. Include details about the data source, data cleaning steps, data transformations, parameter settings, and missing value representation. This documentation will help others (and your future self) understand and reproduce your results.
-
Consider File Size: When transferring large CSV files, consider compression techniques (e.g., gzip) to reduce file size and transfer time. This is particularly important for sharing data over the internet.
By carefully considering these best practices, you can ensure that your CSV writing process is efficient, reliable, and reproducible, leading to more robust and trustworthy data analysis.
Video: Master CSV Writing in R: The Ultimate Step-by-Step Guide
FAQs: Mastering CSV Writing in R
Here are some frequently asked questions about writing CSV files in R, to help clarify the process and ensure you're creating clean, usable data exports.
What's the most basic way to write a dataframe to CSV in R?
The write.csv()
function is the fundamental tool. Simply use write.csv(dataframe_name, "filename.csv", row.names = FALSE)
. The row.names = FALSE
argument prevents writing row numbers to the CSV. This is the most common method to write csv in r.
Can I write a CSV file without column names in R?
Yes, you can. Use the col.names = FALSE
argument within the write.csv()
function. For example: write.csv(dataframe_name, "filename.csv", row.names = FALSE, col.names = FALSE)
. This is useful for specific applications where column names are not required.
How do I handle different separators when writing a CSV?
R defaults to commas as separators but you can modify this. The sep
argument allows you to specify a different separator, like a semicolon: write.csv(dataframe_name, "filename.csv", sep = ";", row.names = FALSE)
. Handling different separators is important for compatibility with various software and regional settings when you write csv in r.
What's the best way to deal with special characters or quotes in my data when writing to CSV?
The quote
argument in write.csv()
controls how strings containing special characters (like commas or quotes) are handled. The default is TRUE
, which encloses strings in double quotes. You can set it to FALSE
(quote = FALSE
) but be aware that this might lead to issues if your data contains the separator character. Using the quote
option correctly is essential for data integrity when you write csv in r.